java -- 网络编程
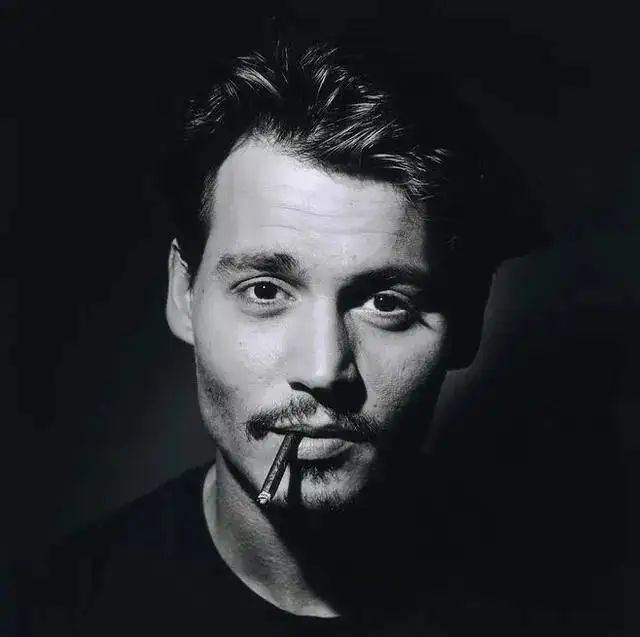
相关标签:
网络编程&UDP
概述
不同的主机可以通过网络通信。
通信三要素:
- 地址ipv4/ipv6, 设备在网络的标识
- 端口 -- 应用程序的标识(自己用1024以上)
- 通信协议 udp/tcp
网络编程-Inetaddress类
InetAddress ip = InetAddress.getByName("server-pc");
System.out.println(ip.getHostAddress());
System.out.println(ip.getHostName());
通信协议
UDP
- 用户数据报协议(User Datagram Protocol)
- 无连接的通信协议,速度快速,一次最多发送64K,不安全,易丢失
发送端
- 找码头
- 打包
- 码头发送
- 付钱走人
public static void main(String[] args) throws IOException {
// 找码头
DatagramSocket datagramSocket = new DatagramSocket();
// 打包
byte[] goodSmoke = "一条好烟".getBytes();
// 在快递上贴上地址
InetAddress address = InetAddress.getByName("localhost");
int port = 10000;
DatagramPacket datagramPacket = new DatagramPacket(goodSmoke,
goodSmoke.length, address, port);
// 发送
datagramSocket.send(datagramPacket);
// 付钱走人
datagramSocket.close();
}
接收端
- 找码头
- 新的箱子
- 从码头接收礼物放入新箱子
- 从箱子中取礼物
- 拿完走人
public static void main(String[] args) throws IOException {
// 找码头
DatagramSocket datagramSocket = new DatagramSocket(10000);
// 新箱子
byte[] byts = new byte[1024];
DatagramPacket dp = new DatagramPacket(byts, byts.length);
// 收礼物,放箱子
datagramSocket.receive(dp);
// 从箱子中拿礼物
String goodSmoke = new String(byts, dp.getOffset()
, dp.getLength());
System.out.println(goodSmoke);
// 走人
datagramSocket.close();
}
UDP-三种通讯方式
- 单播 点对点 之前的代码就是单播
- 组播 一对多 接收端放到一组里
- 广播 一对所有
UDP-组播代码实现
组播地址:
- 224.0.0.0 ~ 239.255.255.255,其中224.0.0.0- 224.0.0.255 为预留的组播地址
发送流程
- 找码头
- 打包
- 码头发送 -- 指定组播地址
- 付钱走人
public class GroupUDPSend {
public static void main(String[] args) throws IOException {
// 1
DatagramSocket ds = new DatagramSocket();
// 打包
byte[] bytes = "hello 组播".getBytes();
InetAddress addrsss = InetAddress.getByName("224.0.1.0");
int port = 10000;
DatagramPacket dp = new DatagramPacket(bytes, bytes.length, addrsss, port);
// 发送
ds.send(dp);
// 4
ds.close();
}
}
接收流程:
- new MulticastSocket(10086)
- 新的箱子
- 把当前电脑添加到组当中
- 从码头接收礼物放入新箱子
- 从箱子中取礼物
- 拿完走人
public class GroupUDPRecv {
public static void main(String[] args) throws IOException {
MulticastSocket ms = new MulticastSocket(10000);
DatagramPacket dp = new DatagramPacket(
new byte[1024], 1024);
// 把当前ip加入组
ms.joinGroup(InetAddress.getByName("224.0.1.0"));
ms.receive(dp);
String s = new String(dp.getData(),
dp.getOffset(), dp.getLength());
System.out.println(s);
ms.close();
}
}
UDP-广播代码实现
一对所有,流程
- 找码头
- 打包 -- 指定广播地址(255.255.255.255) 4个255
- 码头发送
- 付钱走人
public class BroadcastSend {
public static void main(String[] args) throws IOException {
DatagramSocket ds = new DatagramSocket();
byte[] bytes = "广播".getBytes();
DatagramPacket dp = new DatagramPacket(bytes, bytes.length,
InetAddress.getByName("255.255.255.255"), 10000);
ds.send(dp);
ds.close();
}
}
接收端和单播一样
public class MyNetworkRecv {
public static void main(String[] args) throws IOException {
// 找码头
DatagramSocket datagramSocket = new DatagramSocket(10000);
// 新箱子
byte[] byts = new byte[1024];
DatagramPacket dp = new DatagramPacket(byts, byts.length);
// 收礼物,放箱子
datagramSocket.receive(dp);
// 从箱子中拿礼物
String goodSmoke = new String(byts, dp.getOffset(),
dp.getLength());
System.out.println(goodSmoke);
// 走人
datagramSocket.close();
}
}
TCP通讯程序
TCP-客户端
- sc = Socket(host, port)
- sc.getOutputStream()
- 写
- sc.close()
public class TCPClient {
public static void main(String[] args) throws IOException {
Socket sc = new Socket("localhost", 10000);
OutputStream os = sc.getOutputStream();
os.write("hello".getBytes());
sc.close();
}
}
TCP-服务器
- ss = new ServerSocket(port)
- Sockect sc = sc.accept();
- sc.getInputStream()
- sc.close()
- ss.close()
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(11000);
Socket sc = ss.accept();
InputStream is = sc.getInputStream();
int b = 0;
while (((b = is.read()) != -1)) {
System.out.print((char)b);
}
System.out.println();
sc.close();
ss.close();
}
}
服务器与客户端通信
UUID
- UUID.randomUUID().toString() -- 唯一文件名
client:
public class Client {
public static void main(String[] args) throws IOException {
Socket sc = new Socket("localhost", 12000);
OutputStreamWriter outputStreamWriter = new OutputStreamWriter(sc.getOutputStream());
InputStreamReader inputStreamReader = new InputStreamReader(sc.getInputStream());
BufferedWriter bwr = new BufferedWriter(outputStreamWriter);
BufferedReader brr = new BufferedReader(inputStreamReader);
bwr.write("你好啊");
bwr.newLine();
bwr.flush();
System.out.println(brr.readLine());
sc.close();
}
}
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket ss = new ServerSocket(12000);
// 线程池
ExecutorService execService = Executors.newCachedThreadPool();
while (true) {
Socket client = ss.accept();
execService.submit(new MySocketCliekt(client));
}
}
private static class MySocketCliekt implements Runnable, Closeable {
private final BufferedReader bufferedReader;
private final BufferedWriter bufferedWriter;
private final Socket client;
public MySocketCliekt(Socket _client) {
this.client = _client;
try {
OutputStreamWriter outputStreamWriter = new OutputStreamWriter(client.getOutputStream());
InputStreamReader inputStreamReader = new InputStreamReader(client.getInputStream());
bufferedWriter = new BufferedWriter(outputStreamWriter);
bufferedReader = new BufferedReader(inputStreamReader);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void run() {
try {
String s = bufferedReader.readLine();
System.out.println(s);
bufferedWriter.write(s);
bufferedWriter.newLine();
bufferedWriter.flush();
} catch (IOException ignored) {
}
finally {
try {
close();
} catch (IOException ignored) {
}
}
}
@Override
public void close() throws IOException {
try {
bufferedWriter.close();
} finally {
try {
bufferedReader.close();
} finally {
client.close();
}
}
}
}
}
文章来源: https://blog.51cto.com/u_12072082/5687910
特别声明:以上内容(图片及文字)均为互联网收集或者用户上传发布,本站仅提供信息存储服务!如有侵权或有涉及法律问题请联系我们。
举报